CSocketServer
CSocketServer
📢 A collection of classes for creating a socket server, client and communicating between them. Includes a Packetizer to solve the age old issue of TCP/IP packets being fragmented or combined in the pipe.
Must include WS2_32.LIB and/or WSOCK32.LIB
Name | Prototype | Return | Description |
---|---|---|---|
OnAccept | bool OnAccept(CSocketServer *pSock, CSocketClient *pClient) | Return TRUE to allow the connection, FALSE to reject. | Called when a connection is accepted by the socket server. |
OnAcceptConnect | bool OnAcceptConnect(CSocketServer *pSock, CSocketClient *pClient) | Return TRUE to allow the connection, FALSE to reject. | Called when a connection is accepted and/or connected by the socket server. |
OnAfterDePacketize | bool OnAfterDePacketize(CSocketServer *pSock, CSocketClient *pClient, LPBASICHUNK pChunk) | Return TRUE to accept the packet, FALSE to reject. | Called after a packet is read by user code, pChunk contains the actual data sent by the remote peer. |
OnAfterPacketize | bool OnAfterPacketize(CSocketServer *pSock, CSocketClient *pClient, LPBASICHUNK pChunk) | Return TRUE to add to the send queue, FALSE to reject. | Called after a packet is assembled by the socket server, pChunk contains the packet data and the data to be sent. |
OnBeforeDePacketize | bool OnBeforeDePacketize(CSocketServer *pSock, CSocketClient *pClient, LPBASICHUNK pChunk) | Return TRUE to accept the packet, FALSE to reject. | Called before a packet is read by user code, pChunk contains the data sent by the remote peer. |
OnBeforePacketize | bool OnBeforePacketize(CSocketServer *pSock, CSocketClient *pClient, LPBASICHUNK pChunk) | Return TRUE to add to the send queue, FALSE to reject. | Called before a packet is assembled by the socket server, pChunk contains the data to be sent. |
OnConnect | bool OnConnect(CSocketServer *pSock, CSocketClient *pClient) | Return TRUE to allow the connection, FALSE to reject. | Called when a connection is connected by the socket server. |
OnRecv | bool OnRecv(CSocketServer *pSock, CSocketClient *pClient, LPBASICHUNK pChunk) | Return TRUE to accept the data, FALSE to reject. | Called after a packet or partial packet is received, pChunk may not contain a full packet. |
OnSend | bool OnSend(CSocketServer *pSock, CSocketClient *pClient, LPBASICHUNK pChunk) | Return TRUE to send the data, FALSE to delay sending. | Called before a packet is sent, pChunk contains the packet and actual data, guaranteed to be a full packet. |
OnStart | bool OnStart(CSocketServer *pSock, int iListenPort) | Return TRUE to start, FALSE to cancel. | Called when the socket server is started by a call to Start(). |
OnStop | bool OnStop(CSocketServer *pSock) | Return TRUE to stop, FALSE to cancel. | Called when the socket server is stopped by a call to Stop(). |
ClientHandlerThread | void ClientHandlerThread(CSocketServer *pSock, CSocketClient *pClient, LPBASICHUNK pChunk) | n/a | Called when a client is accepted/connected, server enters "one thread per connection" mode, thread exits = client disconnect. |
OnBeginClientThread | void OnBeginClientThread(CSocketServer *pSock, CSocketClient *pClient, HANDLE hThread) | n/a | Called after a client thread is created, before ClientHandlerThread is called. |
OnDisconnect | void OnDisconnect(CSocketServer *pSock, CSocketClient *pClient) | n/a | Called when a client has been disconnected (after socket shutdown). |
OnEndClientThread | void OnEndClientThread(CSocketServer *pSock, CSocketClient *pClient) | n/a | Called after a client thread has been destroyed. |
OnError | void OnError(CSocketServer *pSock, CSocketClient *pClient, int iErrorNumber, const char *sErrorMsg) | n/a | Called for any internal exceptions. |
Running Example
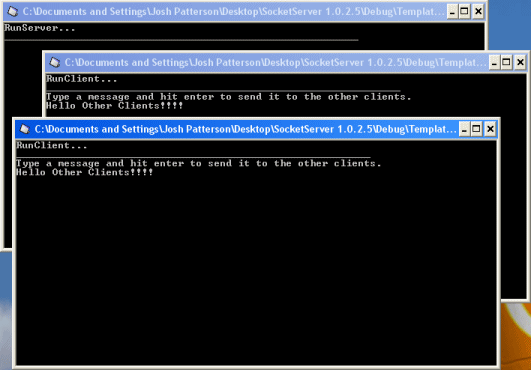